dcmri.AortaLiver2scan#
- class dcmri.AortaLiver2scan(kinetics='1I-IC-D', stationary=None, sequence='SS', free=None, **params)[source]#
Joint model for aorta and liver signals measured over two scans.
This model uses a whole-body model to simultaneously predict signals in aorta and liver, measured over two separate scans.
For more detail on the whole-body model, see Whole body. For more detail on the liver model, see Liver.
- Parameters:
kinetics (str, optional) – Tracer-kinetic liver model. See table Kinetic models for the liver for options - only single-inlet models are allowed. Defaults to ‘1I-IC-D’.
stationary (str, optional) – For intracellular tracers - stationarity regime of the hepatocytes. The options are ‘UE’, ‘E’, ‘U’ or None. For more detail see Liver. Defaults to ‘UE’.
stationary – Stationarity regime of the hepatocytes. The options are ‘UE’, ‘E’, ‘U’ or None. For more detail see Liver. Defaults to ‘UE’.
sequence (str, optional) – imaging sequence. Possible values are ‘SS’ and ‘SR’. Defaults to ‘SS’.
free (dict, optional) – Dictionary with free parameters and their bounds. If not provided, a default set of free parameters is used. Defaults to None.
params (dict, optional) – values for the parameters of the tissue, specified as keyword parameters. Defaults are used for any that are not provided. See tables Aorta-Liver 2-scan parameters and Aorta-Liver 2-scan parameter defaults for a list of parameters and their default values.
See also
Example
Use the model to reconstruct concentrations from experimentally derived signals.
>>> import matplotlib.pyplot as plt >>> import dcmri as dc
Use
fake_tissue
to generate synthetic test data from experimentally-derived concentrations:>>> time, aif, roi, gt = dc.fake_tissue2scan(R10=1/dc.T1(3.0,'liver'))
Since this model generates four time curves, the x- and y-data are tuples:
>>> xdata = (time[0], time[1], time[0], time[1]) >>> ydata = (aif[0], aif[1], roi[0], roi[1])
Build an aorta-liver model and parameters to match the conditions of the fake tissue data:
>>> model = dc.AortaLiver2scan( ... dt = 0.5, ... weight = 70, ... agent = 'gadodiamide', ... dose = 0.2, ... dose2 = 0.2, ... rate = 3, ... field_strength = 3.0, ... t0 = 10, ... TR = 0.005, ... FA = 15, ... )
Train the model on the data:
>>> model.train(xdata, ydata, xtol=1e-3)
Plot the reconstructed signals and concentrations and compare against the experimentally derived data:
>>> model.plot(xdata, ydata)
We can also have a look at the model parameters after training:
>>> model.print_params(round_to=3) ----------------------------------------- Free parameters with their errors (stdev) ----------------------------------------- Bolus arrival time (BAT): 17.13 (1.771) sec Cardiac output (CO): 208.547 (9.409) mL/sec Heart-lung mean transit time (Thl): 12.406 (2.137) sec Heart-lung transit time dispersion (Dhl): 0.459 (0.04) Organs mean transit time (To): 30.912 (3.999) sec Extraction fraction (Eb): 0.064 (0.032) Liver extracellular mean transit time (Te): 2.957 (0.452) sec Liver extracellular dispersion (De): 1.0 (0.146) Liver extracellular volume fraction (ve): 0.077 (0.007) mL/cm3 Hepatocellular uptake rate (khe): 0.002 (0.001) mL/sec/cm3 Hepatocellular transit time (Th): 600.0 (1173.571) sec Organs extraction fraction (Eo): 0.2 (0.057) Organs extracellular mean transit time (Toe): 87.077 (56.882) sec Hepatocellular uptake rate (final) (khe_f): 0.001 (0.001) mL/sec/cm3 Hepatocellular transit time (final) (Th_f): 600.0 (623.364) sec ------------------ Derived parameters ------------------ Blood precontrast T1 (T10a): 1.629 sec Mean circulation time (Tc): 43.318 sec Liver precontrast T1 (T10l): 0.752 sec Biliary excretion rate (kbh): 0.002 mL/sec/cm3 Hepatocellular tissue uptake rate (Khe): 0.023 mL/sec/cm3 Biliary tissue excretion rate (Kbh): 0.002 mL/sec/cm3 Hepatocellular uptake rate (initial) (khe_i): 0.003 mL/sec/cm3 Hepatocellular transit time (initial) (Th_i): 600.0 sec Hepatocellular uptake rate variance (khe_var): 0.934 Biliary tissue excretion rate variance (Kbh_var): 0.0 Biliary excretion rate (initial) (kbh_i): 0.002 mL/sec/cm3 Biliary excretion rate (final) (kbh_f): 0.002 mL/sec/cm3
(
Source code
,png
,hires.png
,pdf
)Notes
Table Aorta-Liver parameters lists the parameters that are relevant in each regime. Table Aorta-Liver parameter defaults list all possible parameters and their default settings.
Aorta-Liver 2-scan parameters# Parameters
When to use
Further detail
dt, tmax
Always
Time axis for forward model
t0, dose_tolerance
Always
For estimating baseline signal
field_strength, weight, agent, dose, dose2, rate
Always
Injection protocol
R10a, R102a, R10l, R102l, S0a, S02a, S0l, S02l
Always
Precontrast R1 (Relaxation model parameters) and S0 (Sequence parameters) for aorta and liver
FA, TR, TS
Always
TC
If sequence is ‘SR’
BAT, BAT2, CO, Thl, Dhl, To, Eo, Tie, Eb
Always
H, ve, De
Always
khe, khe_i, kh_f, Th, Th_i, Th_f
Depends on stationary
Aorta-Liver 2-scan parameter defaults# Parameter
Type
Value
Bounds
Free/Fixed
Simulation
dt
Simulation
0.5
[0, inf]
Fixed
tmax
Simulation
120
[0, inf]
Fixed
dose_tolerance
Simulation
0.1
[0, 1]
Fixed
Injection
field_strength
Injection
3
[0, inf]
Fixed
weight
Injection
70
[0, inf]
Fixed
agent
Injection
‘gadoxetate’
None
Fixed
dose
Injection
0.0125
[0, inf]
Fixed
rate
Injection
1
[0, inf]
Fixed
Signal
R10a
Signal
0.7
[0, inf]
Fixed
R10l
Signal
0.7
[0, inf]
Fixed
S0a
Signal
1
[0, inf]
Free
S0l
Signal
1
[0, inf]
Free
Sequence
FA
Sequence
15
[0, inf]
Fixed
S0
Sequence
1
[0, inf]
Fixed
TC
Sequence
0.1
[0, inf]
Fixed
TR
Sequence
0.005
[0, inf]
Fixed
TS
Sequence
0
[0, inf]
Fixed
Whole body
BAT
Whole body
1200
[0, inf]
Free
CO
Whole body
100
[0, inf]
Free
Thl
Whole body
10
[0, 30]
Free
Dhl
Whole body
0.2
[0.05, 0.95]
Free
To
Whole body
20
[0, 60]
Free
Eo
Whole body
0.15
[0, 0.5]
Free
Toe
Whole body
120
[0, 800]
Free
Eb
Whole body
0.05
[0.01, 0.15]
Free
Liver
H
Kinetic
0.45
[0, 1]
Fixed
Te
Kinetic
30
[0.1, 60]
Free
De
Kinetic
0.85
[0, 1]
Free
ve
Kinetic
0.3
[0.01, 0.6]
Free
khe
Kinetic
0.003
[0, 0.1]
Free
khe_i
Kinetic
0.003
[0, 0.1]
Free
khe_f
Kinetic
0.003
[0, 0.1]
Free
Th
Kinetic
1800
[600, 36000]
Free
Th_i
Kinetic
1800
[600, 36000]
Free
Th_f
Kinetic
1800
[600, 36000]
Free
vol
Kinetic
1000
[0, 10000]
Free
Methods
conc
([sum])Concentrations in aorta and liver.
cost
(xdata, ydata[, metric])Return the goodness-of-fit
Return model parameters with their descriptions
load
([file, path, filename])Load the saved state of the model
params
(*args[, round_to])Return the parameter values
plot
(xdata, ydata[, ref, xlim, fname, show])Plot the model fit against data
predict
(xdata)Predict the data at given time points
print_params
([round_to])Print the model parameters and their uncertainties
relax
()Relaxation rates in aorta and liver.
save
([file, path, filename])Save the current state of the model
set_free
([pop])Set the free model parameters.
train
(xdata, ydata, **kwargs)Train the free parameters
- cost(xdata: tuple, ydata: tuple, metric='NRMS') float [source]#
Return the goodness-of-fit
- Parameters:
xdata (tuple) – tuple of 4 arrays with time points for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The four arrays can be different in length and value.
ydata (tuple) – tuple of 4 arrays with signals for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The arrays can be different in length but each has to have the same length as its corresponding array of time points.
metric (str, optional) – Which metric to use (see notes for possible values). Defaults to ‘NRMS’.
- Returns:
goodness of fit.
- Return type:
Notes
Available options are:
‘RMS’: Root-mean-square.
‘NRMS’: Normalized root-mean-square.
‘AIC’: Akaike information criterion.
‘cAIC’: Corrected Akaike information criterion for small models.
‘BIC’: Baysian information criterion.
- export_params() list #
Return model parameters with their descriptions
- Returns:
Dictionary with one item for each model parameter. The key is the parameter symbol (short name), and the value is a 4-element list with [parameter name, value, unit, sdev].
- Return type:
- load(file=None, path=None, filename='Model')#
Load the saved state of the model
- Parameters:
file (str, optional) – complete path of the file. If this is not provided, a file is constructure from path and filename variables. Defaults to None.
path (str, optional) – path to store the state if file is not provided. Thos variable is ignored if file is provided. Defaults to current working directory.
filename (str, optional) – filename to store the state if file is not provided. If no extension is included, the extension ‘.pkl’ is automatically added. This variable is ignored if file is provided. Defaults to ‘Model’.
- Returns:
class instance
- Return type:
- params(*args, round_to=None)#
Return the parameter values
- plot(xdata: tuple, ydata: tuple, ref=None, xlim=None, fname=None, show=True)[source]#
Plot the model fit against data
- Parameters:
xdata (tuple) – tuple of 4 arrays with time points for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The four arrays can be different in length and value.
ydata (tuple) – tuple of 4 arrays with signals for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The arrays can be different in length but each has to have the same length as its corresponding array of time points.
xlim (array_like, optional) – 2-element array with lower and upper boundaries of the x-axis. Defaults to None.
ref (tuple, optional) – Tuple of optional test data in the form (x,y), where x is an array with x-values and y is an array with y-values. Defaults to None.
fname (path, optional) – Filepath to save the image. If no value is provided, the image is not saved. Defaults to None.
show (bool, optional) – If True, the plot is shown. Defaults to True.
- predict(xdata: tuple) tuple [source]#
Predict the data at given time points
- Parameters:
xdata (tuple) – tuple of 4 arrays with time points for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The four arrays can be different in length and value.
- Returns:
tuple of 4 arrays with signals for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The arrays have the same length as its corresponding array of time points.
- Return type:
- print_params(round_to=None)#
Print the model parameters and their uncertainties
- Parameters:
round_to (int, optional) – Round to how many digits. If this is not provided, the values are not rounded. Defaults to None.
- relax()[source]#
Relaxation rates in aorta and liver.
- Returns:
time points, aorta blood R1, liver R1.
- Return type:
- save(file=None, path=None, filename='Model')#
Save the current state of the model
- Parameters:
file (str, optional) – complete path of the file. If this is not provided, a file is constructure from path and filename variables. Defaults to None.
path (str, optional) – path to store the state if file is not provided. Thos variable is ignored if file is provided. Defaults to current working directory.
filename (str, optional) – filename to store the state if file is not provided. If no extension is included, the extension ‘.pkl’ is automatically added. This variable is ignored if file is provided. Defaults to ‘Model’.
- Returns:
class instance
- Return type:
- set_free(pop=None, **kwargs)#
Set the free model parameters.
- Parameters:
pop (str or list) – a single variable or a list of variables to remove from the list of free parameters.
- Raises:
ValueError – if the pop argument contains a parameter that is not in the list of free parameters.
ValueError – If the parameter is not a model parameter, or bounds are not properly formatted.
- train(xdata: tuple, ydata: tuple, **kwargs)[source]#
Train the free parameters
- Parameters:
xdata (tuple) – tuple of 4 arrays with time points for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The four arrays can be different in length and value.
ydata (tuple) – tuple of 4 arrays with signals for aorta in the first scan, aorta in the second stand, liver in the first scan, and liver in the second scan, in that order. The arrays can be different in length but each has to have the same length as its corresponding array of time points.
kwargs – any keyword parameters accepted by
scipy.optimize.curve_fit
.
- Returns:
A reference to the model instance.
- Return type:
Examples using dcmri.AortaLiver2scan
#
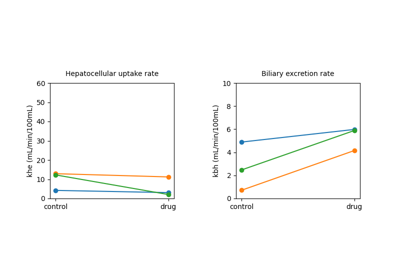
Clinical - rifampicin effect in subjects with impaired liver function
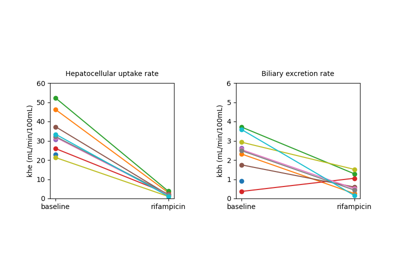
Clinical - rifampicin induced inhibition of liver function